標(biāo)題:《C語言實現(xiàn)實時顯示時間與空間占用:技術(shù)解析與應(yīng)用》
在計算機科學(xué)領(lǐng)域,實時顯示程序運行的時間和空間占用情況對于性能分析和調(diào)試至關(guān)重要。本文將深入探討如何利用C語言實現(xiàn)這一功能,并通過實際代碼示例展示其應(yīng)用。
一、引言
實時顯示程序運行的時間和空間占用情況,有助于開發(fā)者了解程序性能,優(yōu)化算法,提高代碼質(zhì)量。在C語言編程中,我們可以通過系統(tǒng)調(diào)用和編程技巧來實現(xiàn)這一功能。
二、實時顯示時間
在C語言中,我們可以使用clock()
函數(shù)來獲取程序運行的時間。clock()
函數(shù)返回程序開始運行到當(dāng)前時刻所消耗的CPU時鐘周期數(shù)。為了方便顯示,我們需要將其轉(zhuǎn)換為秒。
#include <stdio.h>
#include <time.h>
int main() {
clock_t start, end;
double cpu_time_used;
start = clock();
// 程序運行代碼
end = clock();
cpu_time_used = ((double) (end - start)) / CLOCKS_PER_SEC;
printf("程序運行時間:%f秒\n", cpu_time_used);
return 0;
}
三、實時顯示空間占用
在C語言中,我們可以通過計算程序運行前后的內(nèi)存占用差值來獲取空間占用情況。這需要借助操作系統(tǒng)提供的內(nèi)存分配函數(shù),如malloc()
和free()
。
#include <stdio.h>
#include <stdlib.h>
int main() {
void *ptr1, *ptr2;
size_t space_used;
ptr1 = malloc(10 * sizeof(int));
if (ptr1 == NULL) {
printf("內(nèi)存分配失敗\n");
return -1;
}
ptr2 = malloc(20 * sizeof(int));
if (ptr2 == NULL) {
printf("內(nèi)存分配失敗\n");
free(ptr1);
return -1;
}
free(ptr1);
free(ptr2);
space_used = (size_t)(ptr2 - ptr1);
printf("空間占用:%zu字節(jié)\n", space_used);
return 0;
}
四、綜合示例
以下是一個綜合示例,展示了如何同時顯示時間和空間占用情況。
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
clock_t start, end;
double cpu_time_used;
void *ptr1, *ptr2;
size_t space_used;
start = clock();
ptr1 = malloc(10 * sizeof(int));
ptr2 = malloc(20 * sizeof(int));
cpu_time_used = ((double) (end - start)) / CLOCKS_PER_SEC;
space_used = (size_t)(ptr2 - ptr1);
printf("程序運行時間:%f秒\n", cpu_time_used);
printf("空間占用:%zu字節(jié)\n", space_used);
free(ptr1);
free(ptr2);
return 0;
}
五、總結(jié)
本文介紹了如何利用C語言實現(xiàn)實時顯示時間和空間占用情況。通過系統(tǒng)調(diào)用和編程技巧,我們可以方便地獲取程序性能數(shù)據(jù),為優(yōu)化算法和調(diào)試代碼提供有力支持。在實際開發(fā)過程中,這一功能具有很高的實用價值。
轉(zhuǎn)載請注明來自衡水悅翔科技有限公司,本文標(biāo)題:《《C語言實現(xiàn)實時顯示時間與空間占用:技術(shù)解析與應(yīng)用》》
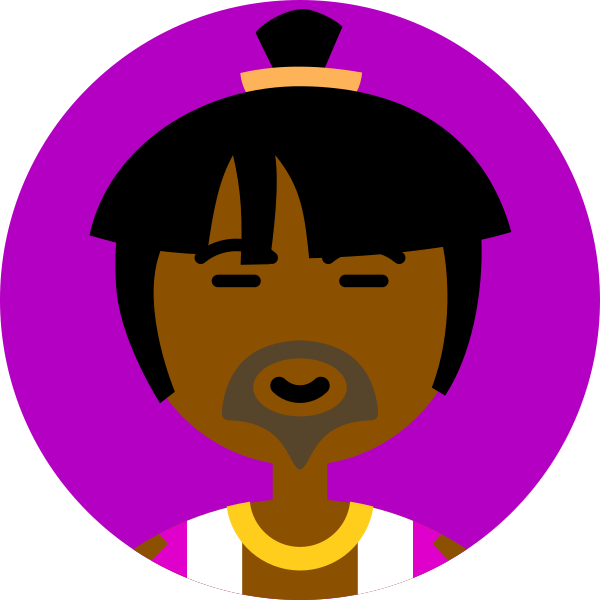